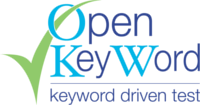 |
OpenKeyWord
Build_ID: 457, Datum: 01.02.2020 07:45:48
Dont repeat yourself. - Do it once and only once!
|
40 package okw.gui.adapter.selenium.webdriver;
42 import java.util.ArrayList;
44 import okw.exceptions.OKWFrameObjectMethodNotImplemented;
45 import okw.gui.adapter.selenium.SeAnyChildWindow;
85 ArrayList<String> lvLsReturn =
new ArrayList<String>();
117 Boolean lvbReturn =
true;
143 Boolean lvbReturn =
true;
146 this.LogPrintDebug(
"Allways true" );
161 Boolean lvbReturn =
false;
189 ArrayList<String> lvLsReturn =
new ArrayList<String>();
218 ArrayList<String> lvLsReturn =
new ArrayList<String>();
246 ArrayList<String> lvLsReturn =
new ArrayList<String>();
366 public void TypeKey(ArrayList<String> fps_Values)
void SetFocus()
Setzt den Focus in das aktuelle URL-TextFeld.
void TypeKey(ArrayList< String > fps_Values)
Setzt den Wert der URL im aktiven Browser/BrowserChild und navigiert zur gegebenen Seite.
Boolean getHasFocus()
Methode wird nicht untertütz, Methode liefert den aktuellen Zustand Wert des Focus.
void SetValue(ArrayList< String > fps_Values)
Setzt den Wert der URL im aktiven Browser und navigiert zur gegebene Seite.
void LogFunctionEndDebug()
Methode ruft die Methode Logger.Instance.LogFunctionEndDebug() auf.
Die Ausnahme OKWFrameObjectMethodNotImplemented wird ausgelöst, wenn im Frame Objekt eine gegebene Me...
ArrayList< String > getValue()
Alle Value Schlüsselwörter werden nicht unterstützt.
static SeDriver getInstance()
Gibt die Instance für die einzige Instanz dieser Klasse zurück.
Boolean getExists()
Prüft die Existenz des aktuellen Objektes.
Boolean getIsActive()
Ermittelt, ob das aktuellen Objekt aktiv ist.
ArrayList< String > getCaption()
Ermittelt den textuellen Inhalt der Überschrift eines HTML-Tags anhand des Attributee "textContent".
ArrayList< String > getLabel()
Ermittelt den textuellen Inhalt des Labels.
void ClickOn()
Klickt auf das aktuelle Objekt.
ArrayList< String > getTooltip()
Liest den aktuellen Tooltip-Wert der URL aus.
void LogFunctionStartDebug(String fpsMethodName)
Methode ruft die Methode Logger.Instance.LogFunctionStartDebug(fps_FunctionName), und erweitert den g...