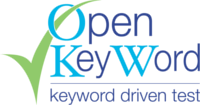 |
OpenKeyWord
Build_ID: 457, Datum: 01.02.2020 07:45:48
Dont repeat yourself. - Do it once and only once!
|
40 package okw.gui.adapter.selenium;
42 import java.util.ArrayList;
44 import okw.LogMessenger;
45 import okw.OKW_Const_Sngltn;
46 import okw.exceptions.OKWNotAllowedValueException;
125 super(Locator, Locators);
149 Boolean lvbReturn =
false;
156 lvbReturn = this.
Me().isSelected();
215 ArrayList<String> lvls_Return =
new ArrayList<String>();
226 lvls_Return.add(lvsValue);
231 lvls_Return.add(lvsValue);
267 if ( fps_Values.get(0).equalsIgnoreCase( lvsCHECKED ) )
271 else if (fps_Values.get(0).equalsIgnoreCase( lvsUNCHECKED ) )
278 String lvsLM = this.LM.
GetMessage(
"Common",
"OKWNotAllowedValueException", fps_Values.get(0));
303 public void Select(ArrayList<String> fps_Values)
316 if (fps_Values.get(0).equalsIgnoreCase( lvsCHECKED ) )
320 else if (fps_Values.get(0).equalsIgnoreCase( lvsUNCHECKED ) )
327 String lvsLM = this.LM.
GetMessage(
"Common",
"OKWNotAllowedValueException", fps_Values.get(0));
Boolean WaitForMe()
Wartet auf das Objekt Wenn kein Fenster gefunden wird,.
LogMessenger liest Log-Meldungen sprachspezifisch für die im Konstruktor gegeben Klasse aus der Zugeh...
String GetConst4Internalname(String fpsInternalname)
Methode ermittelt für Internalname und der aktuell eingestellten Sprache den Wert für Const.
void LogFunctionEndDebug()
Methode ruft die Methode Logger.Instance.LogFunctionEndDebug() auf.
String GetMessage(String MethodName, String TextKey)
Holt die Log-Meldung für MethodeNmae/Textkey ohne weitere Parameter.
static OKW_Const_Sngltn getInstance()
Holt die einzige Instanz dieser Klasse.
OKW_Const verwaltet die sprachabhängigen OKW-Konstanten.
WebElement Me()
Ermittelt aus dem gegebenen Locator das DOM-Elelement, welches das Objekt representiert.
void LogFunctionStartDebug(String fpsMethodName)
Methode ruft die Methode Logger.Instance.LogFunctionStartDebug(fps_FunctionName), und erweitert den g...
OKWNotAllowedValueException-Ausnahme wird ausgelöst, wenn ein gegebener Wert im Schlüsselwort nicht e...
void ClickOn()
Das ist die GUI-Adapter Methode, die durch das Schlüsselwort ClickOn( FN ) aufgerufen wird.