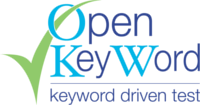 |
OpenKeyWord
Build_ID: 457, Datum: 01.02.2020 07:45:48
Dont repeat yourself. - Do it once and only once!
|
40 package okw.gui.adapter.selenium.webdriver;
42 import java.util.ArrayList;
44 import okw.exceptions.OKWFrameObjectMethodNotImplemented;
45 import okw.gui.adapter.selenium.SeAnyChildWindow;
85 ArrayList<String> lvLsReturn =
new ArrayList<String>();
113 Boolean lvbReturn =
true;
137 Boolean lvbReturn =
false;
166 Boolean lvbReturn =
true;
169 this.LogPrintDebug(
"Allways true" );
188 ArrayList<String> lvLsReturn =
new ArrayList<String>();
217 ArrayList<String> lvLsReturn =
new ArrayList<String>();
246 ArrayList<String> lvLsReturn =
new ArrayList<String>();
352 public void TypeKey(ArrayList<String> fps_Values)
void TypeKey(ArrayList< String > fps_Values)
Setzt den Wert der URL im aktiven Browser/BrowserChild und navigiert zur gegebenen Seite.
void LogFunctionEndDebug()
Methode ruft die Methode Logger.Instance.LogFunctionEndDebug() auf.
ArrayList< String > getTooltip()
Liest den aktuellen Tooltip-Wert der URL aus.
void SetValue(ArrayList< String > fps_Values)
Setzt eines Wert ist nicht möglich.
Die Ausnahme OKWFrameObjectMethodNotImplemented wird ausgelöst, wenn im Frame Objekt eine gegebene Me...
static SeDriver getInstance()
Gibt die Instance für die einzige Instanz dieser Klasse zurück.
ArrayList< String > getCaption()
Ermittelt den textuellen Inhalt der Überschrift eines HTML-Tags anhand des Attributee "textContent".
Boolean getIsActive()
Ermittelt, ob das aktuellen Objekt aktiv ist.
Boolean getExists()
Prüft die Existenz des aktuellen Objektes.
void LogFunctionStartDebug(String fpsMethodName)
Methode ruft die Methode Logger.Instance.LogFunctionStartDebug(fps_FunctionName), und erweitert den g...
void SetFocus()
Setzt eines Wert ist nicht möglich.
void ClickOn()
Das ist die GUI-Adapter Methode, die durch das Schlüsselwort ClickOn( FN ) aufgerufen wird.
Boolean getHasFocus()
Methode wird nicht untertütz, Methode liefert den aktuellen Zustand Wert des Focus.
ArrayList< String > getLabel()
Ermittelt den textuellen Inhalt des Labels.
ArrayList< String > getValue()
Alle Value Schlüsselwörter werden nicht unterstützt.